Trecco my First iOS app
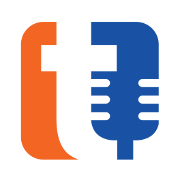
I have not posted in awhile, but I have been busy. I find that this is a recurring theme with my blog. I make a string of posts on time and then I get caught up writing code. Back to the post at hand.
Over the holidays I spent some time to learn Swift and write an iOS app. Trecco was the app that I built. It will record a voice note, use IBM’s Watson to transcribe the voice note, and then save it to a Trello board as a card.